Wallpaper in a NetBeans RCP application
Yesterday I wrote about my attempts to put an image in the root window of a Netbeans RCP application that is shown when no TopComponents are open.
Today I did finalize the code and added so I could add a logotype at the bottom on the right side and when the application window is resized it will stay at the bottom right side. So here is the complete code.
private ImageIcon bgimage;
@Override
public void restored() {
bgimage = new ImageIcon(ImageUtilities.loadImage("path/to/my/wallpaper.jpg"));
SwingUtilities.invokeLater(new Runnable() {
public void run() {
JFrame main = (JFrame) WindowManager.getDefault().getMainWindow();
JPanel mainPanel = new JPanel(new BorderLayout()) {
@Override
public void add(Component comp, Object constraints) {
super.add(comp, constraints);
if (constraints == BorderLayout.CENTER) {
if (comp instanceof JPanel) {
final JPanel panel = (JPanel) comp;
panel.setBorder(BorderFactory.createEmptyBorder());
panel.add(new BackgroundLabel(bgimage), java.awt.BorderLayout.CENTER);
}
}
}
};
main.setContentPane(mainPanel);
}
});
}
}
private class BackgroundLabel extends JLabel {
private boolean showBgImage = true;
private ComponentAdapter componentAdapter;
private Image image;
public BackgroundLabel(ImageIcon imageIcon) {
super(imageIcon);
image = ((ImageIcon) getIcon()).getImage();
setupAdapter();
TopComponent.getRegistry().addPropertyChangeListener(new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
if (TopComponent.getRegistry().getOpened().isEmpty()) {
showBgImage = true;
repaint();
} else {
showBgImage = false;
}
}
});
}
@Override
public void paintComponent(Graphics g) {
if (showBgImage) {
int x = getParent().getSize().width - image.getWidth(this);
int y = getParent().getSize().height - image.getHeight(this);
g.drawImage(image, x, y, image.getWidth(this), image.getHeight(this), this);
}
}
private void setupAdapter() {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
componentAdapter = new ComponentAdapter() {
@Override
public void componentResized(ComponentEvent e) {
BackgroundLabel.this.setSize(getParent().getSize());
}
};
getParent().addComponentListener(componentAdapter);
}
});
}
}
Any image loaded into bgimage will be put at the bottom right corner of the application and stay there even when the window resizes.
To modify this behaviour just change the x and y parameters in paintComponent(Graphics g). For example setting them to 0,0 would draw the image at the top left corner. To stretch an image the width and height parameters should be modified.
Here is two screenshots showing this in action. The first one is just a quick logo I did created in GIMP since I can't show my real application here, the other one is a fullscreen netbeans desktop background downloaded from http://netbeans.org/community/teams/evangelism/collateral.html.
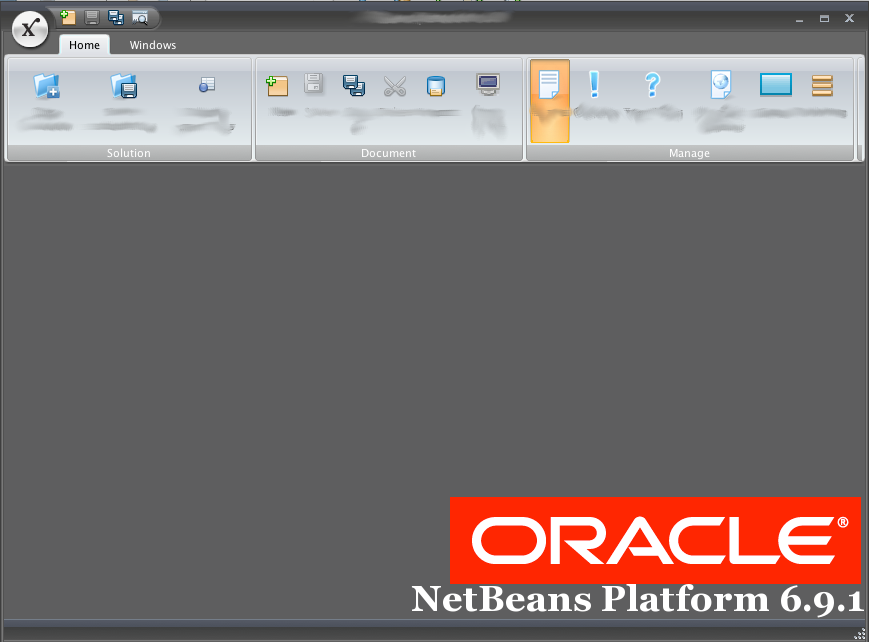
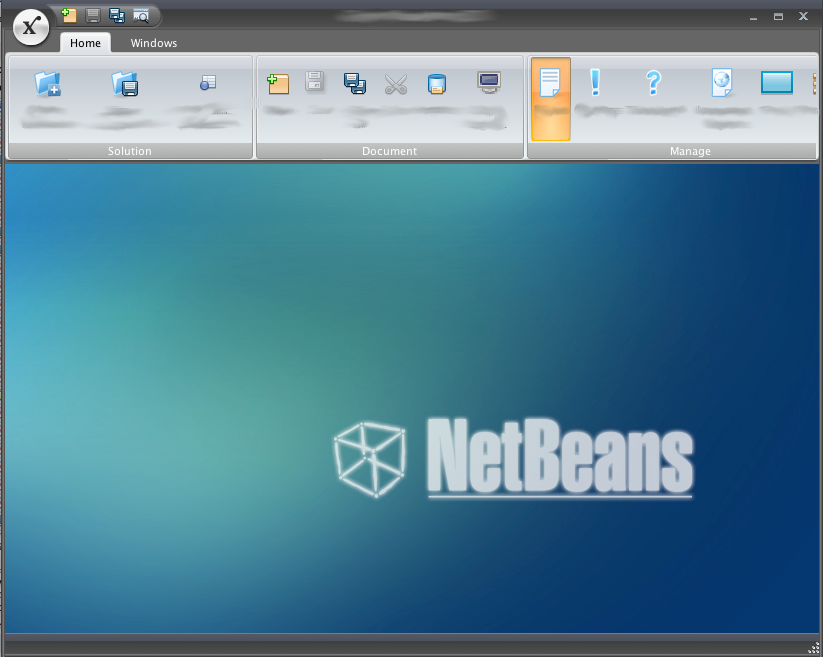